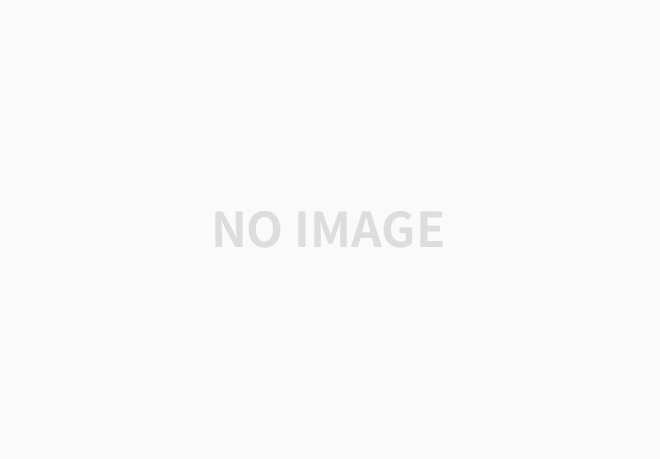
지난번에는 xml 파일을 생성하여 세터 및 생성자를 주입하는 방법을 알아봤습니다.
📝 오늘은 어노테이션(annotation)을 사용하여 객체를 사용할 수 있는 방법을 알아보겠습니다.
@Configuration을 사용하면 Beans 설정이 되는 파일임을 알릴 수 있습니다.
기존에는 xml 파일을 생성하여 빈즈를 읽어 사용했는데, 이번엔 어노테이션으로
불러올 것이기 때문에 "어노테이션을 사용하여 만든 이 객체를 보낼 거야!"를
알리기 위해 @Bean 어노테이션을 사용하여 객체를 생성해야합니다.
✔️ @Configuration 어노테이션은 xml 파일에서 <Beans> 태그이고
✔️ @Bean 어노테이션은 xml 파일에서 <Bean> 태그를 뜻합니다.
예제로 확인해보겠습니다.
📝 간단하게 이름, 나이, 취미를 출력하는 코드를 짜보겠습니다.
✔️ getter 및 setter 생성
package com.ex.spring;
import java.util.ArrayList;
public class Student {
private String name;
private int age;
private ArrayList<String> hobbys;
private double height;
private double weight;
public Student(String name, int age, ArrayList<String> hobbys) {
this.name = name;
this.age = age;
this.hobbys = hobbys;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public ArrayList<String> getHobbys() {
return hobbys;
}
public void setHobbys(ArrayList<String> hobbys) {
this.hobbys = hobbys;
}
public double getHeight() {
return height;
}
public void setHeight(double height) {
this.height = height;
}
public double getWeight() {
return weight;
}
public void setWeight(double weight) {
this.weight = weight;
}
}
기존에 xml 파일에 작성했던 <Beans> 태그와 <Bean> 태그를
java 클래스에서 사용하려면 아래와 같이 어노테이션을 붙여 사용하시면 됩니다.
✔️ @Configuration, @Beans, @Bean 어노테이션
학생 두 명의 이름, 나이, 취미를 설정합니다
package com.ex.spring;
import java.util.ArrayList;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class ApplicationConfig {
@Bean
public Student student1() {
ArrayList<String> hobbys = new ArrayList<String>();
hobbys.add("수영");
hobbys.add("요리");
Student student = new Student("홍길동", 20, hobbys);
student.setHeight(180);
student.setWeight(80);
return student;
}
@Bean
public Student student2() {
ArrayList<String> hobbys = new ArrayList<String>();
hobbys.add("독서");
hobbys.add("음악감상");
Student student = new Student("홍길순", 18, hobbys);
student.setHeight(170);
student.setWeight(55);
return student;
}
}
이제 메인 클래스에서는 해당 어노테이션을 인식해야 합니다.
✔️ 메인 클래스에서 AnnotationConfigApplicationContext를 사용하여
@Beans와 @Bean으로 설정한 객체를 읽을 수 있도록 합니다.
이렇게 설정하면 자동으로 annotation bean을 사용하여 만든 객체를 읽을 수 있게 되고
✔️ getBean을 사용하면 해당 객체를 불러올 수 있습니다.
package com.ex.spring;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
public class Main {
public static void main(String[] args) {
AnnotationConfigApplicationContext ctx = new AnnotationConfigApplicationContext(ApplicationConfig.class);
Student student1 = ctx.getBean("student1", Student.class);
System.out.println("이름: " + student1.getName());
System.out.println("나이: " + student1.getAge());
System.out.println("취미: " + student1.getHobbys());
System.out.println("신장: " + student1.getHeight());
System.out.println("몸무게: " + student1.getWeight());
Student student2 = ctx.getBean("student2", Student.class);
System.out.println("이름: " + student1.getName());
System.out.println("나이: " + student1.getAge());
System.out.println("취미: " + student1.getHobbys());
System.out.println("신장: " + student1.getHeight());
System.out.println("몸무게: " + student1.getWeight());
ctx.close();
}
}
저는 여기서 실행할 때 아래와 같은 에러가 떴습니다.

root of context hierarchy Exception in thread "main" java.lang.IllegalStateException:
CGLIB is required to process @Configuration classes.
Either add CGLIB to the classpath or remove the following @Configuration bean definitions: [applicationConfig]
요약하자면 CGLIB 라이브러리가 없다는 뜻입니다. CGLIB 는 Java 클래스를 조작하는 라이브러리로,
스프링에서 @Configuration 어노테이션을 처리하기 위해서 필요한 라이브러리입니다.
Maven이나 Gradle 등 프로젝트 의존성 관리 도구를 사용하여 CGLIB를 추가하는 방법이 있으나
저는 Maven이나 Gradle 프로젝트를 사용하지 않으므로... pom.xml 파일에 아래와 같은 코드를 추가했습니다.
<!-- https://mvnrepository.com/artifact/cglib/cglib -->
<dependency>
<groupId>cglib</groupId>
<artifactId>cglib</artifactId>
<version>3.2.0</version>
</dependency>
<!-- https://mvnrepository.com/artifact/cglib/cglib-nodep -->
<dependency>
<groupId>cglib</groupId>
<artifactId>cglib-nodep</artifactId>
<version>3.2.0</version>
<scope>test</scope>
</dependency>
아무데나 추가했더니 <dependency>는 부모가 있어야 한다고 합니다.
보니까 다른 모든 <dependency>는 <project> 안에 있더라고요.
그래서 저는 가장 마지막 <dependency> 아래에 해당 코드를 추가했습니다.
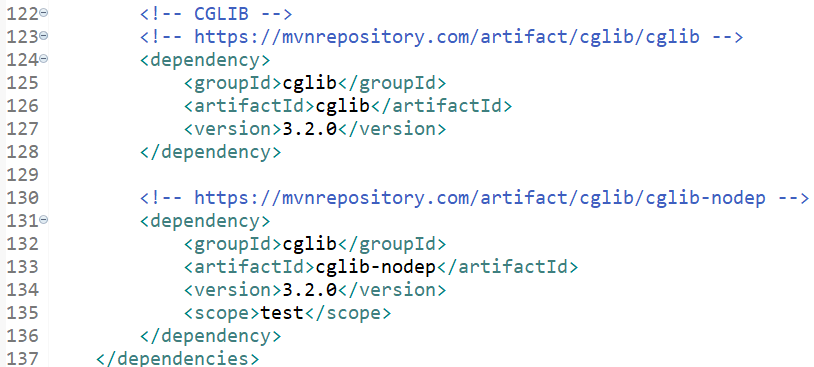
이 코드를 추가하면 CGLIB 라이브러리 에러가 더이상 뜨지 않는 것을 확인하실 수 있습니다.
📝 xml에서 context:annotation-config 사용하기
✔️ 메인클래스에서 student1은 남기고 student2는 삭제
package com.ex.spring;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
public class Main {
public static void main(String[] args) {
AnnotationConfigApplicationContext ctx = new AnnotationConfigApplicationContext(ApplicationConfig.class);
Student student1 = ctx.getBean("student1", Student.class);
System.out.println("이름: " + student1.getName());
System.out.println("나이: " + student1.getAge());
System.out.println("취미: " + student1.getHobbys());
System.out.println("신장: " + student1.getHeight());
System.out.println("몸무게: " + student1.getWeight());
ctx.close();
}
}
✔️ xml 파일 생성하여 student2 객체 추가
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="student2" class="com.ex.spring.Student">
<constructor-arg value="홍길동" />
<constructor-arg value="30" />
<constructor-arg>
<list>
<value>마라톤</value>
<value>요리</value>
</list>
</constructor-arg>
<property name="height" value="190" />
<property name="weight" value="70" />
</bean>
</beans>
xml에서 annotation을 사용한 java 파일을 불러와 사용하겠다는 걸 알리기 위해
✔️ <context:annotation-config />를 추가합니다.
<context:annotation-config />
<bean class="com.ex.spring.ApplicationConfig" />
추가하면 처음에 빨간줄에 뜰 텐데
✔️ Namespace에서 context를 추가하면 (체크) 에러가 사라집니다.
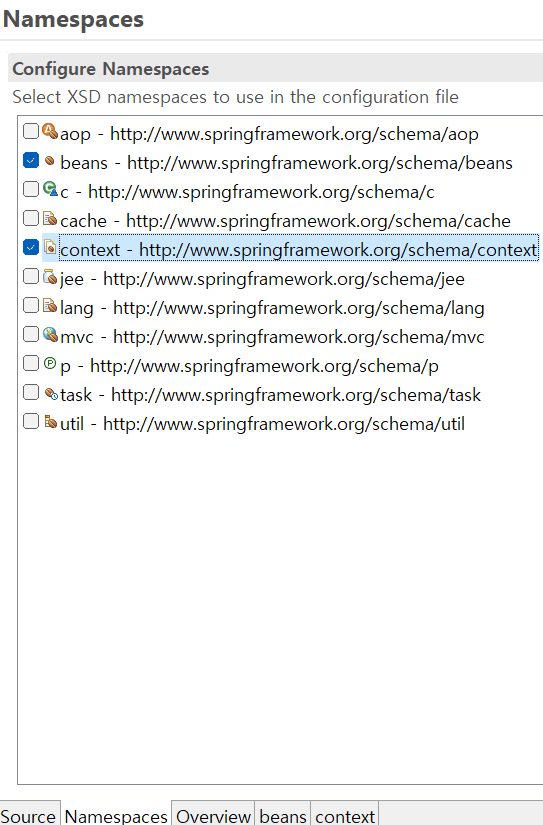
메인클래스
package com.ex.spring;
import org.springframework.context.support.AbstractApplicationContext;
import org.springframework.context.support.GenericXmlApplicationContext;
public class Main {
public static void main(String[] args) {
AbstractApplicationContext ctx = new GenericXmlApplicationContext("classpath:applicationCTX/xml");
Student student1 = ctx.getBean("student1", Student.class);
System.out.println("이름: " + student1.getName());
System.out.println("나이: " + student1.getAge());
System.out.println("취미: " + student1.getHobbys());
System.out.println("신장: " + student1.getHeight());
System.out.println("몸무게: " + student1.getWeight());
Student student2 = ctx.getBean("student2", Student.class);
System.out.println("이름: " + student2.getName());
System.out.println("나이: " + student2.getAge());
System.out.println("취미: " + student2.getHobbys());
System.out.println("신장: " + student2.getHeight());
System.out.println("몸무게: " + student2.getWeight());
ctx.close();
}
}
'2023-02 몰입형 SW 정규 교육' 카테고리의 다른 글
[SpringBoot] 네이버 번역 API 사용하기 (파파고) (1) | 2023.12.05 |
---|---|
[Spring] 이클립스(eclipse) XML 기반 세터/생성자 주입 (setter/constructor) (1) | 2023.10.23 |
[Spring] 이클립스(eclipse) 자바 스프링 프로젝트 생성 방법 (0) | 2023.10.23 |
[Vue] VS Code에 Vue 프로젝트 생성 (0) | 2023.09.22 |
HTTP 상태 코드 100 ~ 500 (0) | 2023.09.21 |